Laravelでのcollectionをすっきり整理!データ操作方法を解説
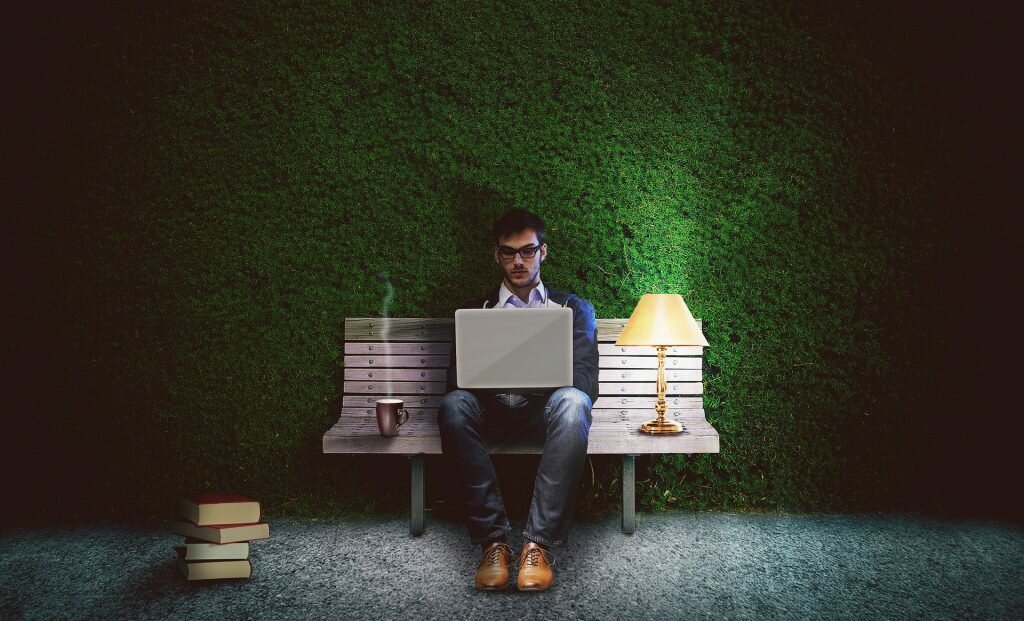
- システム
エンジニア - collectionの使い方について教えてください。
- プロジェクト
マネージャー - collectionとは配列に機能を加えたもので、データ取得・集計などができます。
Laravelでのcollectionの使い方とは?
今回は、Laravelでのcollectionの使い方について説明します。collectionとは、配列に機能を加えたもので、様々なデータ操作が行えます。
ここでは、collectionを使った
・データ取得
・データ集計
・フィルタ
・ソート
について紹介します。
Laravelでのcollectionの使い方に興味のある方はぜひご覧ください。
データ取得
Laravelでのcollectionを使ったデータ取得について紹介します。
・all()
要素をすべて取得します。
1
2
|
$collection = collect(['item1', 'item2', 'item3', 'item4', 'item5']);
print_r($collection->all());
|
実行結果は以下のようになります。
1
|
Array ( [0] => item1 [1] => item2 [2] => item3 [3] => item4 [4] => item5 )
|
・first()
先頭要素を取得します。
1
2
|
$collection = collect(['item1', 'item2', 'item3', 'item4', 'item5']);
echo $collection->first();
|
実行結果は以下のようになります。
1
|
item1
|
・last()
末尾の要素を取得します。
1
2
|
$collection = collect(['item1', 'item2', 'item3', 'item4', 'item5']);
echo $collection->last();
|
実行結果は以下のようになります。
1
|
item5
|
・get()
インデックス指定で取得します。
1
2
|
$collection = collect(['item1', 'item2', 'item3', 'item4', 'item5']);
echo $collection->get(2);
|
実行結果は以下のようになります。
1
|
item3
|
このような使い方もできます。
1
2
3
4
5
6
|
$collection = collect([
'id' => 1,
'name' => 'taro',
'age' => 20
]);
echo $collection->get('name');
|
実行結果は以下のようになります。
1
|
taro
|
・take()
指定要素数分取得します。
1
2
3
|
$collection = collect(['item1', 'item2', 'item3', 'item4', 'item5']);
$chunk = $collection->take(3);
print_r($chunk->toArray());
|
実行結果は以下のようになります。
1
|
Array ( [0] => item1 [1] => item2 [2] => item3 )
|
負値を指定すると、末尾から指定要素数分取得します。
1
2
3
|
$collection = collect(['item1', 'item2', 'item3', 'item4', 'item5']);
$chunk = $collection->take(-3);
print_r($chunk->toArray());
|
実行結果は以下のようになります。
1
|
Array ( [2] => item3 [3] => item4 [4] => item5 )
|
データ集計
Laravelでのcollectionを使ったデータ集計について紹介します。
・count
要素数を取得します。
1
2
|
$collection = collect(['item1', 'item2', 'item3', 'item4', 'item5']);
echo $collection->count();
|
実行結果は以下のようになります。
1
|
5
|
・average()
平均値を取得します。
1
2
|
$collection = collect([1, 2, 3, 4, 5]);
echo $collection->avg();
|
実行結果は以下のようになります。
1
|
3
|
・max()
最大値を取得します。
1
2
|
$collection = collect([1, 2, 3, 4, 5]);
echo $collection->max();
|
実行結果は以下のようになります。
1
|
5
|
・min()
最小値を取得します。
1
2
|
$collection = collect([1, 2, 3, 4, 5]);
echo $collection->min();
|
実行結果は以下のようになります。
1
|
1
|
・sum()
合計値を取得します。
1
2
|
$collection = collect([1, 2, 3, 4, 5]);
echo $collection->sum();
|
実行結果は以下のようになります。
1
|
15
|
フィルタ
Laravelでのcollectionを使ったフィルタについて紹介します。
・where()
条件に一致する要素を取得します。
1
2
3
4
5
6
7
8
9
|
$collection = collect([
['id' => 1, 'name' => 'ichiro', 'age' => 15],
['id' => 2, 'name' => 'jiro', 'age' => 20],
['id' => 3, 'name' => 'saburo', 'age' => 25],
['id' => 4, 'name' => 'shiro', 'age' => 30],
['id' => 5, 'name' => 'goro', 'age' => 25]
]);
$filtered = $collection->where('age', 25);
print_r($filtered->toArray());
|
実行結果は以下のようになります。
1
|
Array ( [2] => Array ( [id] => 3 [name] => saburo [age] => 25 ) [4] => Array ( [id] => 5 [name] => goro [age] => 25 ) )
|
・whereIn()
複数条件に一致する要素を取得します。
1
2
3
4
5
6
7
8
9
|
$collection = collect([
['id' => 1, 'name' => 'ichiro', 'age' => 15],
['id' => 2, 'name' => 'jiro', 'age' => 20],
['id' => 3, 'name' => 'saburo', 'age' => 25],
['id' => 4, 'name' => 'shiro', 'age' => 30],
['id' => 5, 'name' => 'goro', 'age' => 25]
]);
$filtered = $collection->whereIn('age', [20, 25]);
print_r($filtered->toArray());
|
実行結果は以下のようになります。
1
|
Array ( [1] => Array ( [id] => 2 [name] => jiro [age] => 20 ) [2] => Array ( [id] => 3 [name] => saburo [age] => 25 ) [4] => Array ( [id] => 5 [name] => goro [age] => 25 ) )
|
・whereNotIn()
複数条件に一致しない要素を取得します。
1
2
3
4
5
6
7
8
9
|
$collection = collect([
['id' => 1, 'name' => 'ichiro', 'age' => 15],
['id' => 2, 'name' => 'jiro', 'age' => 20],
['id' => 3, 'name' => 'saburo', 'age' => 25],
['id' => 4, 'name' => 'shiro', 'age' => 30],
['id' => 5, 'name' => 'goro', 'age' => 25]
]);
$filtered = $collection->whereNotIn('age', [20, 25]);
print_r($filtered->toArray());
|
実行結果は以下のようになります。
1
|
Array ( [0] => Array ( [id] => 1 [name] => ichiro [age] => 15 ) [3] => Array ( [id] => 4 [name] => shiro [age] => 30 ) )
|
・filter()
フィルタ条件に一致した要素を取得します。
1
2
3
4
5
|
$collection = collect([1, 2, 3, 4, 5]);
$filtered = $collection->filter(function ($val, $key) {
return $val > 2;
});
print_r($filtered->all());
|
実行結果は以下のようになります。
1
|
Array ( [2] => 3 [3] => 4 [4] => 5 )
|
フィルタ条件に一致しない要素を取得します。
1
2
3
4
5
|
$collection = collect([1, 2, 3, 4, 5]);
$filtered = $collection->reject(function ($val, $key) {
return $val > 2;
});
print_r($filtered->all());
|
実行結果は以下のようになります。
1
|
Array ( [0] => 1 [1] => 2 )
|
このように、Laravelではcollectionを使ってフィルタできます。
ソート
Laravelでのcollectionを使ったソートについて紹介します。
・sort()
要素を昇順ソートします。
1
2
3
|
$collection = collect([30, 24, 37, 4, 48]);
$sorted = $collection->sort();
print_r($sorted->toArray());
|
実行結果は以下のようになります。
1
|
Array ( [3] => 4 [1] => 24 [0] => 30 [2] => 37 [4] => 48 )
|
・sortDesc()
要素を降順ソートします。
1
2
3
|
$collection = collect([30, 24, 37, 4, 48]);
$sorted = $collection->sortDesc();
print_r($sorted->toArray());
|
実行結果は以下のようになります。
1
|
Array ( [4] => 48 [2] => 37 [0] => 30 [1] => 24 [3] => 4 )
|
・sortBy()
特定のカラムで昇順ソートします。
1
2
3
4
5
6
7
8
9
|
$collection = collect([
['id' => 1, 'name' => 'ichiro', 'age' => 15],
['id' => 2, 'name' => 'jiro', 'age' => 20],
['id' => 3, 'name' => 'saburo', 'age' => 25],
['id' => 4, 'name' => 'shiro', 'age' => 30],
['id' => 5, 'name' => 'goro', 'age' => 25]
]);
$sorted = $collection->sortBy('age');
print_r($sorted->toArray());
|
実行結果は以下のようになります。
1
|
Array ( [0] => Array ( [id] => 1 [name] => ichiro [age] => 15 ) [1] => Array ( [id] => 2 [name] => jiro [age] => 20 ) [2] => Array ( [id] => 3 [name] => saburo [age] => 25 ) [4] => Array ( [id] => 5 [name] => goro [age] => 25 ) [3] => Array ( [id] => 4 [name] => shiro [age] => 30 ) )
|
このように、Laravelではcollectionを使ってソートできます。
- システム
エンジニア - collectionを使ってフィルタやソートもできるのですね。
- プロジェクト
マネージャー - collectionでのデータ操作をマスターしましょう。
まとめ
いかがでしたでしょうか。Laravelでのcollectionの使い方について説明しました。collectionとは、配列に機能を加えたもので、様々なデータ操作が行えます。
ここで紹介した以外にも、様々なcollectionの関数があります。
ぜひご自身でLaravelのソースコードを書いて、理解を深めてください。
FEnet.NETナビ・.NETコラムは株式会社オープンアップシステムが運営しています。
株式会社オープンアップシステムはこんな会社です
秋葉原オフィスには株式会社オープンアップシステムをはじめグループのIT企業が集結!
数多くのエンジニアが集まります。

-
スマホアプリから業務系システムまで
スマホアプリから業務系システムまで開発案件多数。システムエンジニア・プログラマーとしての多彩なキャリアパスがあります。
-
充実した研修制度
毎年、IT技術のトレンドや社員の要望に合わせて、カリキュラムを刷新し展開しています。社内講師の丁寧なサポートを受けながら、自分のペースで学ぶことができます。
-
資格取得を応援
スキルアップしたい社員を応援するために資格取得一時金制度を設けています。受験料(実費)と合わせて資格レベルに合わせた最大10万円の一時金も支給しています。
-
東証プライム上場企業グループ
オープンアップシステムは東証プライム上場「株式会社夢真ビーネックスグループ」のグループ企業です。
安定した経営基盤とグループ間のスムーズな連携でコロナ禍でも安定した雇用を実現させています。
株式会社オープンアップシステムに興味を持った方へ
株式会社オープンアップシステムでは、開発系エンジニア・プログラマを募集しています。
年収をアップしたい!スキルアップしたい!大手の上流案件にチャレンジしたい!
まずは話だけでも聞いてみたい場合もOK。お気軽にご登録ください。
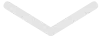
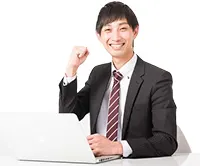
新着案件New Job
-
開発エンジニア/東京都品川区/【WEB面談可】/在宅ワーク
月給29万~30万円東京都品川区(大崎駅) -
遠隔テストサービス機能改修/JavaScript/東京都港区/【WEB面談可】/テレワーク
月給45万~60万円東京都港区(六本木駅) -
病院内システムの不具合対応、保守/東京都豊島区/【WEB面談可】/テレワーク
月給30万~30万円東京都豊島区(池袋駅) -
開発/JavaScript/東京都豊島区/【WEB面談可】/テレワーク
月給50万~50万円東京都豊島区(大塚駅) -
債権債務システム追加開発/東京都文京区/【WEB面談可】/在宅勤務
月給62万~67万円東京都文京区(後楽園駅) -
PMO/東京都豊島区/【WEB面談可】/在宅勤務
月給55万~55万円東京都豊島区(池袋駅)