C#での画像処理について紹介|グレイスケール変換・明るさ変更を使いこなしましょう
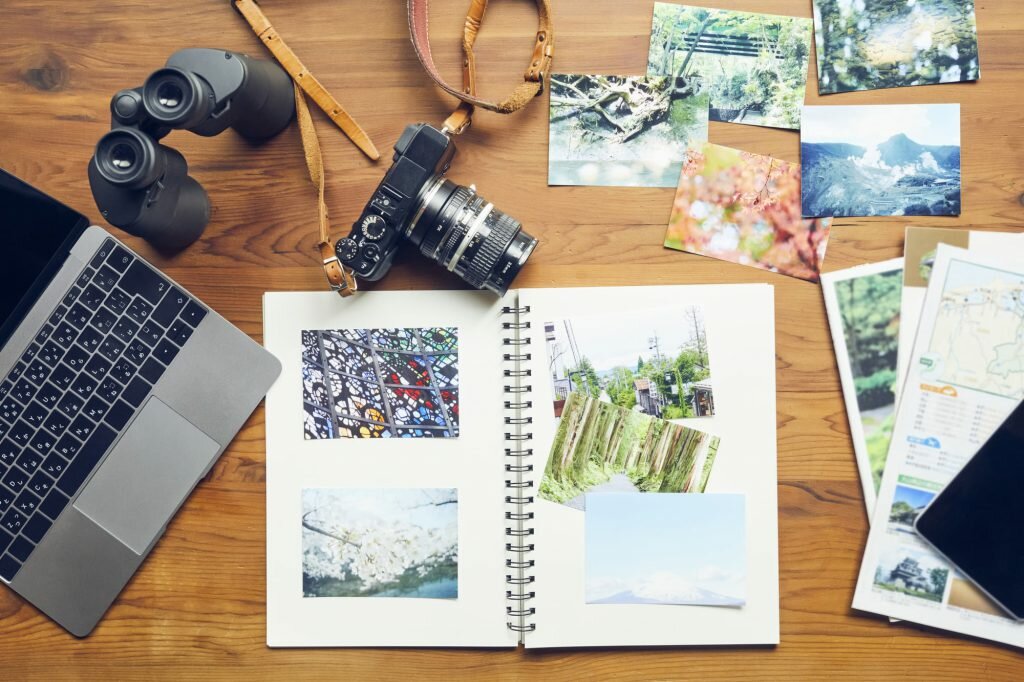
- システム
エンジニア - C#でRBG値の取得や変換方法などの画像処理について教えていただけますか。
- プロジェクト
マネージャー - それでは、C#での画像処理についてご紹介いたしましょう。
C#の画像処理について
今回は、C#の画像処理について説明します。
画像処理の基本は、pixel単位に分解して処理することです。
RBG値の取得や変換方法について紹介します。
C#の画像処理に興味のある方はぜひご覧ください。
RGB値の取得
C#では、pixelのRGB(Red, Green, Blue)値を取得できます。
クリックした座標のRGB値を取得するプログラムを作成します。
実際のソースコードを見てみましょう。
事前に”C:\test\test.jpeg”を準備しておいてください。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
|
using System;
using System.Drawing;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
PictureBox pictureBox;
Bitmap bitmap;
public Form1()
{
this.AutoSize = true;
this.Load += Form1_Load;
}
private void Form1_Load(object sender, EventArgs e)
{
bitmap = new Bitmap(@"C:\test\test.jpeg");
pictureBox = new PictureBox();
pictureBox.Location = new Point(10, 10);
pictureBox.SizeMode = PictureBoxSizeMode.AutoSize;
pictureBox.Image = bitmap;
pictureBox.MouseDown += PictureBox_MouseDown;
this.Controls.Add(pictureBox);
}
private void PictureBox_MouseDown(object sender, MouseEventArgs e)
{
Color color = bitmap.GetPixel(e.X, e.Y);
Console.WriteLine(color.R);
Console.WriteLine(color.G);
Console.WriteLine(color.B);
}
}
}
|
画像をクリックすると、指定したpixelのRGBが標準出力されることが分かります。
このように、C#ではpixelのRGB(Red, Green, Blue)値を取得できます。
グレイスケール変換
C#で画像をグレイスケール変換してみます。
実際のソースコードを見てみましょう。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
|
using System;
using System.Drawing;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
PictureBox pictureBox1, pictureBox2;
Bitmap bitmap1, bitmap2;
public Form1()
{
this.AutoSize = true;
this.Load += Form1_Load;
}
private void Form1_Load(object sender, EventArgs e)
{
bitmap1 = new Bitmap(@"C:\test\test.jpeg");
pictureBox1 = new PictureBox();
pictureBox1.Location = new Point(10, 10);
pictureBox1.SizeMode = PictureBoxSizeMode.AutoSize;
pictureBox1.Image = bitmap1;
this.Controls.Add(pictureBox1);
bitmap2 = LoadImageGray(@"C:\test\test.jpeg");
pictureBox2 = new PictureBox();
pictureBox2.Location = new Point(10, bitmap1.Height + 20);
pictureBox2.SizeMode = PictureBoxSizeMode.AutoSize;
pictureBox2.Image = bitmap2;
this.Controls.Add(pictureBox2);
}
static Bitmap LoadImageGray(string fileName)
{
Bitmap bitmap = new Bitmap(fileName);
int w = bitmap.Width;
int h = bitmap.Height;
byte[,] data = new byte[w, h];
// bitmapクラスの画像pixel値を配列に挿入
for (int i = 0; i < h; i++)
{
for (int j = 0; j < w; j++)
{
// グレイスケール変換処理
data[j, i] = (byte)((bitmap.GetPixel(j, i).R + bitmap.GetPixel(j, i).B + bitmap.GetPixel(j, i).G) / 3);
bitmap.SetPixel(j, i, Color.FromArgb(data[j, i], data[j, i], data[j, i]));
}
}
return bitmap;
}
}
}
|
pixelをグレイスケールに変換し、BitmapにSetPixelで設定して画像変換しています。
このように、C#で画像をグレイスケール変換してみます。
明るさ変更
C#で画像の明るさを変更できます。
実際のソースコードを見てみましょう。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
|
using System;
using System.Drawing;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
PictureBox pictureBox1, pictureBox2;
Bitmap bitmap1, bitmap2;
public Form1()
{
this.AutoSize = true;
this.Load += Form1_Load;
}
private void Form1_Load(object sender, EventArgs e)
{
bitmap1 = LoadImageGray(@"C:\test\test.jpeg");
pictureBox1 = new PictureBox();
pictureBox1.Location = new Point(10, 10);
pictureBox1.SizeMode = PictureBoxSizeMode.AutoSize;
pictureBox1.Image = bitmap1;
this.Controls.Add(pictureBox1);
bitmap2 = LoadImageGray(@"C:\test\test.jpeg");
bitmap2 = BrightnessChange(bitmap2, 50);
pictureBox2 = new PictureBox();
pictureBox2.Location = new Point(10, bitmap1.Height + 20);
pictureBox2.SizeMode = PictureBoxSizeMode.AutoSize;
pictureBox2.Image = bitmap2;
this.Controls.Add(pictureBox2);
}
static Bitmap LoadImageGray(string fileName)
{
Bitmap bmp = new Bitmap(fileName);
int w = bmp.Width;
int h = bmp.Height;
byte[,] data = new byte[w, h];
// bitmapクラスの画像pixel値を配列に挿入
for (int i = 0; i < h; i++)
{
for (int j = 0; j < w; j++)
{
// グレイスケール変換処理
data[j, i] = (byte)((bmp.GetPixel(j, i).R + bmp.GetPixel(j, i).B + bmp.GetPixel(j, i).G) / 3);
bmp.SetPixel(j, i, Color.FromArgb(data[j, i], data[j, i], data[j, i]));
}
}
return bmp;
}
static Bitmap BrightnessChange(Bitmap bmp, int bright)
{
// 画像データの幅と高さを取得
int w = bmp.Width;
int h = bmp.Height;
byte[,] data = new byte[w, h];
byte[,] brightdata = new byte[w, h];
for (int i = 0; i < h; i++)
{
for (int j = 0; j < w; j++)
{
// bmpのデータを取得
data[j, i] = (byte)((bmp.GetPixel(j, i).R + bmp.GetPixel(j, i).B + bmp.GetPixel(j, i).G) / 3);
// 明るさ変更処理
if ((int)data[j, i] + bright >= 256)
{
brightdata[j, i] = 255;
}
else if ((int)data[j, i] + bright < 0)
{
brightdata[j, i] = 0;
}
else
{
brightdata[j, i] = (byte)(data[j, i] + bright);
}
// bmpに設定
bmp.SetPixel(j, i, Color.FromArgb(brightdata[j, i], brightdata[j, i], brightdata[j, i]));
}
}
return bmp;
}
}
}
|
pixel値を取得して明るさ値を加えています。
256を超えるようであれば、最大値の255になるようにしています。
このように、C#で画像の明るさを変更できます。
色反転(ネガポジ反転)
C#で画像の色反転(ネガポジ反転)ができます。
実際のソースコードを見てみましょう。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
|
using System;
using System.Drawing;
using System.Windows.Forms;
namespace WindowsFormsApp1
{
public partial class Form1 : Form
{
PictureBox pictureBox1, pictureBox2;
Bitmap bitmap1, bitmap2;
public Form1()
{
this.AutoSize = true;
this.Load += Form1_Load;
}
private void Form1_Load(object sender, EventArgs e)
{
bitmap1 = LoadImageGray(@"C:\test\test.jpeg");
pictureBox1 = new PictureBox();
pictureBox1.Location = new Point(10, 10);
pictureBox1.SizeMode = PictureBoxSizeMode.AutoSize;
pictureBox1.Image = bitmap1;
this.Controls.Add(pictureBox1);
bitmap2 = LoadImageGray(@"C:\test\test.jpeg");
bitmap2 = ReverseColor(bitmap2);
pictureBox2 = new PictureBox();
pictureBox2.Location = new Point(10, bitmap1.Height + 20);
pictureBox2.SizeMode = PictureBoxSizeMode.AutoSize;
pictureBox2.Image = bitmap2;
this.Controls.Add(pictureBox2);
}
static Bitmap LoadImageGray(string fileName)
{
Bitmap bmp = new Bitmap(fileName);
int w = bmp.Width;
int h = bmp.Height;
byte[,] data = new byte[w, h];
// bitmapクラスの画像pixel値を配列に挿入
for (int i = 0; i < h; i++)
{
for (int j = 0; j < w; j++)
{
// グレイスケール変換処理
data[j, i] = (byte)((bmp.GetPixel(j, i).R + bmp.GetPixel(j, i).B + bmp.GetPixel(j, i).G) / 3);
bmp.SetPixel(j, i, Color.FromArgb(data[j, i], data[j, i], data[j, i]));
}
}
return bmp;
}
static Bitmap ReverseColor(Bitmap bmp)
{
// 縦横サイズを配列から読み取り
int w = bmp.Width;
int h = bmp.Height;
// 出力画像用の配列
byte[,] src = new byte[w, h];
byte[,] dst = new byte[w, h];
// ネガポジ反転処理
for (int i = 0; i < h; i++)
{
for (int j = 0; j < w; j++)
{
src[j, i] = (byte)((bmp.GetPixel(j, i).R + bmp.GetPixel(j, i).B + bmp.GetPixel(j, i).G) / 3);
dst[j, i] = DoubleToByte(255 - src[j, i]);
bmp.SetPixel(j, i, Color.FromArgb(dst[j, i], dst[j, i], dst[j, i]));
}
}
return bmp;
}
// doubleをbyteに変換
static byte DoubleToByte(double num)
{
if (num > 255.0) return 255;
else if (num < 0) return 0;
else return (byte)num;
}
}
}
|
255 – src[j, i]とすることで、色反転しています。
このように、C#で画像の色反転(ネガポジ反転)ができます。
- システム
エンジニア - C#は画像処理もプログラミングできるのですね。
- プロジェクト
マネージャー - ご紹介したソースコードを参考に、ご自身でソースコードを書いて理解を深めてください。
まとめ
いかがでしたでしょうか。
C#での画像処理について紹介しました。
グレイスケール変換・明るさ変更・色反転(ネガポジ反転)について紹介しました。
ぜひご自身でソースコードを書いて、理解を深めてください。
FEnet.NETナビ・.NETコラムは株式会社オープンアップシステムが運営しています。
株式会社オープンアップシステムはこんな会社です
秋葉原オフィスには株式会社オープンアップシステムをはじめグループのIT企業が集結!
数多くのエンジニアが集まります。

-
スマホアプリから業務系システムまで
スマホアプリから業務系システムまで開発案件多数。システムエンジニア・プログラマーとしての多彩なキャリアパスがあります。
-
充実した研修制度
毎年、IT技術のトレンドや社員の要望に合わせて、カリキュラムを刷新し展開しています。社内講師の丁寧なサポートを受けながら、自分のペースで学ぶことができます。
-
資格取得を応援
スキルアップしたい社員を応援するために資格取得一時金制度を設けています。受験料(実費)と合わせて資格レベルに合わせた最大10万円の一時金も支給しています。
-
東証プライム上場企業グループ
オープンアップシステムは東証プライム上場「株式会社夢真ビーネックスグループ」のグループ企業です。
安定した経営基盤とグループ間のスムーズな連携でコロナ禍でも安定した雇用を実現させています。
株式会社オープンアップシステムに興味を持った方へ
株式会社オープンアップシステムでは、開発系エンジニア・プログラマを募集しています。
年収をアップしたい!スキルアップしたい!大手の上流案件にチャレンジしたい!
まずは話だけでも聞いてみたい場合もOK。お気軽にご登録ください。
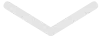
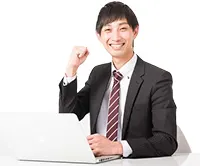
C#新着案件New Job
-
システム開発/東京都新宿区/【WEB面談可/C#経験者/20代前半の方活躍中/経験1年以上の方活躍中】/在宅勤務
月給29万~34万円東京都新宿区(新宿駅) -
システム開発/東京都新宿区/【WEB面談可/C#経験者/20代後半~40代の方活躍中/経験年数不問】/在宅勤務
月給41万~50万円東京都新宿区(新宿駅) -
デバック、テスト項目の作成/神奈川県横浜市/【WEB面談可/C#経験者/20代前半の方活躍中/経験1年以上の方活躍中】/在宅勤務
月給29万~34万円神奈川県横浜市(桜木町駅) -
デバック、テスト項目の作成/神奈川県横浜市/【WEB面談可/C#経験者/20代後半~40代の方活躍中/経験年数不問】/在宅勤務
月給41万~50万円神奈川県横浜市(桜木町駅) -
基幹システム開発導入/東京都新宿区/【WEB面談可/C#経験者/20代前半の方活躍中/経験1年以上の方活躍中】/在宅勤務
月給29万~34万円東京都新宿区(西新宿駅) -
基幹システム開発導入/東京都新宿区/【WEB面談可/C#経験者/20代後半~40代の方活躍中/経験年数不問】/在宅勤務
月給41万~50万円東京都新宿区(西新宿駅)